Mastering the Gas API: A Guide for Developers
A concise guide on how to use the Gas API by MetaMask, a comprehensive tool designed to provide current information on the computational costs of operations on the Ethereum Virtual Machine (EVM).
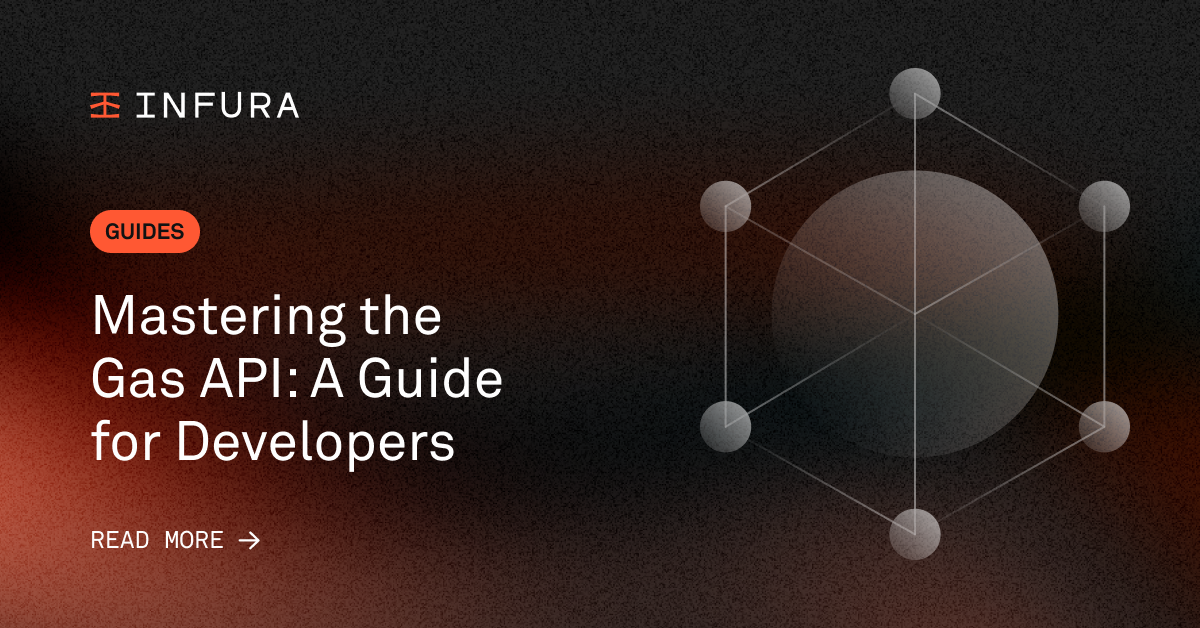
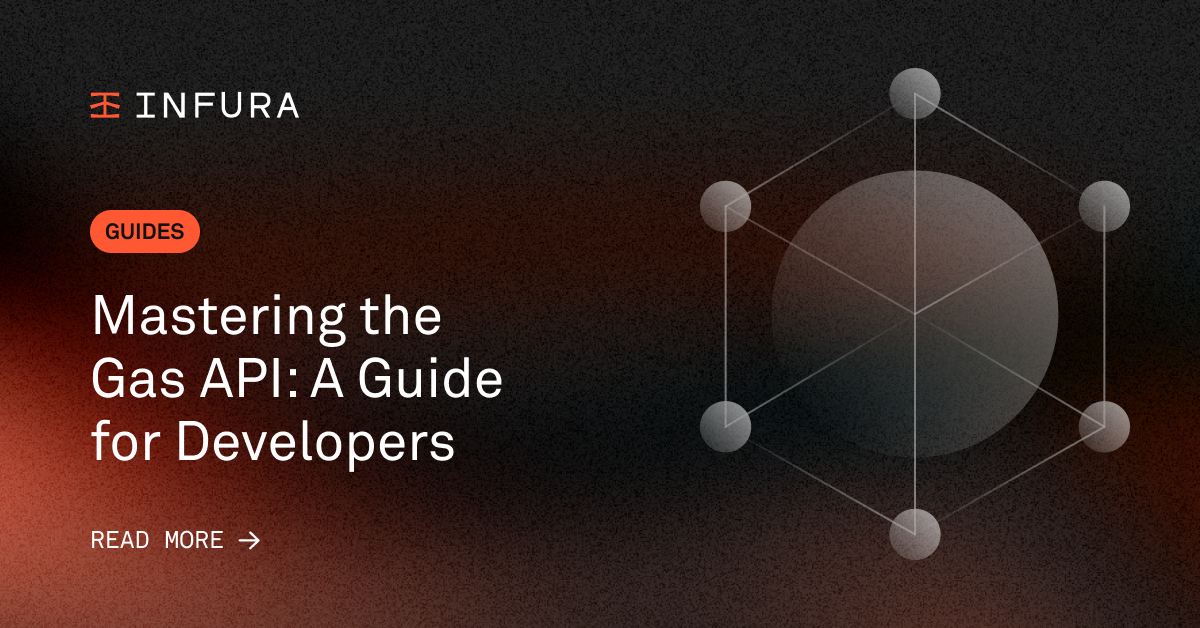
A concise guide on how to use the Gas API by MetaMask, a comprehensive tool designed to provide current information on the computational costs of operations on the Ethereum Virtual Machine (EVM).
In the dynamic world of Ethereum development, understanding and efficiently managing gas prices is crucial. Enter the Gas API – your go-to tool for real-time gas price insights on Ethereum Virtual Machine compatible chains. This guide will walk you through the basics of the Gas API on Infura, its setup, and practical applications.
What is the Gas API?
The Gas API is a comprehensive tool designed to provide current information on the computational costs of operations on Ethereum Virtual Machine (EVM) compatible chains. It's pivotal for optimizing transactions in terms of speed and cost-efficiency. With this API, you can:
- Fetch Real-Time Gas Prices: Like checking current gasoline prices, it helps decide when to perform transactions at lower costs.
- Access Gas Price History: By reviewing past gas prices, developers can identify trends and predict future costs, similar to weather forecasting.
- Estimate Transaction Speed: It provides insights into how quickly transactions might process at different gas prices, aiding users who prioritize speed.
What can you do with the Gas API?
- Get EIP-1559 Gas Prices: It provides the latest gas prices under the EIP-1559 standard, which is vital for modern Ethereum applications needing dynamic fee structures, such as decentralized exchanges or automated transaction services.
- Get the Base Fee History (in GWEI): This offers historical data on base fees, which is important for applications like market analysis platforms or data aggregators in the blockchain space, helping in trend analysis and economic forecasting.
- Get the Base Fee Percentile (in GWEI): It shows the range of fees for past transactions. It is useful in applications where predicting the likelihood and timing of transaction inclusion is key, such as in high-frequency trading on Ethereum.
- Get the Busy Threshold for a Network: This endpoint indicates network congestion levels. It's essential for timing-sensitive applications, like smart contract deployment tools, to schedule transactions during less busy periods, potentially saving on fees and avoiding delays.
Setting up the environment
Before diving in, ensure you have the following prerequisites:
- A valid Web3 API key and API key secret.
- Node.js and npm are installed on your system.
Step 1: Initialize your project
Start by setting up your project directory:
mkdir new_project
cd new_project
npm init -y
Step 2: Install the required packages
Install axios for making HTTP requests and dotenv for managing environment variables:
npm install axios dotenv
Step 3: Configure environment variables
Create a .env file and add your Infura credentials:
INFURA_API_KEY=<your-api-key>
INFURA_API_KEY_SECRET=<your-api-key-secret>
Remember to replace placeholder values with your actual Infura credentials.
Step 4: Writing the script
Create an index.js file and add the following code snippet:
const axios = require("axios");
require("dotenv").config();
const Auth = Buffer.from(
process.env.INFURA_API_KEY + ":" + process.env.INFURA_API_KEY_SECRET,
).toString("base64");
const chainId = 1; // Ethereum Mainnet
(async () => {
try {
const { data } = await axios.get(
`https://gas.api.infura.io/networks/${chainId}/suggestedGasFees`,
{
headers: { Authorization: `Basic ${Auth}` },
},
);
console.log("Suggested gas fees:", data);
} catch (error) {
console.log("Server responded with:", error);
}
})();
This script will fetch the current suggested gas fees for Ethereum Mainnet.
Step 5: Run your script
Execute your script with:
node index.js
Get started using the Gas API
The steps outlined in this guide provide a solid foundation for integrating the Gas API into your Ethereum-based projects whether you're maintaining legacy systems or developing modern decentralized applications, the Gas API is an indispensable tool in your developer toolkit.
For a deeper dive into the functionalities, additional features, and advanced applications of the Gas API, check out the Gas API documentation.